Bootstrap Form
Bootstrap offers a wide range of classes and components to style and enhance forms easily.
Bootstrap Form Component
Below Listed most common component of form.
Bootstrap Form control
-
Documentation URL : https://getbootstrap.com/docs/5.3/forms/form-control/
-
Code Snippet :
<div class="mb-3">
<label for="firstname" class="form-label">First Name</label>
<input type="text" class="form-control" id="firstname">
</div>
-
Here
-
mb-3 : Used for margin bottom 1rem
-
form-label : Use for label
-
form-control : Use for input
-
Bootstrap Form Select
-
Documentation URL : https://getbootstrap.com/docs/5.3/forms/select/
-
Code Snippet :
<div class="mb-3">
<label for="country" class="form-label">Country</label>
<select class="form-select" id="country">
<option selected disabled>Select Country</option>
<option value="bangladesh">Bangladesh</option>
<option value="japan">Japan</option>
<option value="chine">Chine</option>
<option value="not-listed">Not Listed</option>
</select>
</div>
-
Here
-
mb-3 : Used for margin bottom 1rem
-
form-label : Use for label
-
form-select : Use for select
-
Bootstrap Form checkbox
-
Documentation URL : https://getbootstrap.com/docs/5.3/forms/checks-radios/
-
Code Snippet :
<div class="form-check">
<input class="form-check-input" type="checkbox" value="" id="laptop">
<label class="form-check-label" for="laptop">
Laptop
</label>
</div>
-
Here
-
form-check : Checkbox wrapper class
-
form-check-label : Manage the label left of the input
-
form-check-input : Used in Checkbox input
-
Bootstrap Form radio
-
Documentation URL : https://getbootstrap.com/docs/5.3/forms/checks-radios/
-
Code Snippet :
<div class="form-check">
<input class="form-check-input" type="radio" name="sex" id="male">
<label class="form-check-label" for="male">
Male
</label>
</div>
-
Here
-
form-check : Radio wrapper class
-
form-check-label : Manage the label left of the input
-
form-check-input : Used in Radio input
-
Bootstrap Form range input
-
Documentation URL : https://getbootstrap.com/docs/5.3/forms/range
-
Code Snippet :
<div class="mb-3">
<label for="work-hours" class="form-label">Work Hours</label>
<input type="range" class="form-range" id="work-hours">
</div>
-
Here
-
mb-3 : Used for margin bottom 1rem
-
form-label : Use for label
-
form-range : Use for range input
-
Bootstrap Form color picker
-
Documentation URL : https://getbootstrap.com/docs/5.3/forms/form-control/#color
-
Code Snippet :
<div class="mb-3">
<label for="color-picker" class="form-label">Color picker</label>
<input type="color" class="form-control form-control-color" id="color-picker">
</div>
-
Here
-
mb-3 : Used for margin bottom 1rem
-
form-label : Use for label
-
form-control-color : Use for color input
-
Example of Bootstrap Form
-
Documentation URL : https://getbootstrap.com/docs/5.3/forms/overview/
-
Code Snippet :
<div class="row">
<div class="col-md-6">
<div class="mb-3">
<label for="firstname" class="form-label">First Name</label>
<input type="text" class="form-control" id="firstname">
</div>
</div>
<div class="col-md-6">
<div class="mb-3">
<label for="lastname" class="form-label">Last Name</label>
<input type="text" class="form-control" id="lastname">
</div>
</div>
<div class="col-md-6">
<div class="mb-3">
<label for="email" class="form-label">Email Address</label>
<input type="email" class="form-control" id="email">
</div>
</div>
<div class="col-md-6">
<div class="mb-3">
<label for="password" class="form-label">Password</label>
<input type="password" class="form-control" id="password">
</div>
</div>
<div class="col-md-6">
<div class="mb-3">
<label for="country" class="form-label">Country</label>
<select class="form-select" id="country">
<option selected disabled>Select Country</option>
<option value="bangladesh">Bangladesh</option>
<option value="japan">Japan</option>
<option value="chine">Chine</option>
<option value="not-listed">Not Listed</option>
</select>
</div>
</div>
<div class="col-md-6">
<label for="username" class="form-label">Username</label>
<div class="input-group mb-3">
<span class="input-group-text" id="basic-addon1">@</span>
<input type="text" id="username" class="form-control" placeholder="Username">
</div>
</div>
<div class="col-md-12">
<div class="mb-3">
<label for="work-hours" class="form-label">Work Hours</label>
<input type="range" class="form-range" id="work-hours">
</div>
</div>
<div class="col-md-4">
<div class="form-check">
<input class="form-check-input" type="checkbox" value="" id="laptop">
<label class="form-check-label" for="laptop">
Laptop
</label>
</div>
<div class="form-check">
<input class="form-check-input" type="checkbox" value="" id="mobile">
<label class="form-check-label" for="mobile">
Mobile
</label>
</div>
<div class="form-check">
<input class="form-check-input" type="checkbox" value="" id="power-bank">
<label class="form-check-label" for="power-bank">
Power Bank
</label>
</div>
<div class="form-check">
<input class="form-check-input" type="checkbox" value="" id="ips-backup">
<label class="form-check-label" for="ips-backup">
IPS Backup
</label>
</div>
</div>
<div class="col-md-4">
<div class="form-check">
<input class="form-check-input" type="radio" name="sex" id="male">
<label class="form-check-label" for="male">
Male
</label>
</div>
<div class="form-check">
<input class="form-check-input" type="radio" name="sex" id="female">
<label class="form-check-label" for="female">
Female
</label>
</div>
<div class="form-check">
<input class="form-check-input" type="radio" name="sex" id="other">
<label class="form-check-label" for="other">
Other
</label>
</div>
<div class="form-check">
<input class="form-check-input" type="radio" name="sex" id="na">
<label class="form-check-label" for="na">
Not Applicable
</label>
</div>
</div>
<div class="col-md-4">
<div class="mb-3">
<label for="color-picker" class="form-label">Color picker</label>
<input type="color" class="form-control form-control-color" id="color-picker">
</div>
</div>
<div class="col-md-12">
<div class="mb-3 mt-3">
<label for="text-area" class="form-label">About</label>
<textarea class="form-control" id="text-area" rows="3"></textarea>
</div>
</div>
<div class="col-md-12 mb-4">
<button type="button" class="btn btn-success">
Register
</button>
</div>
</div>
-
Output :
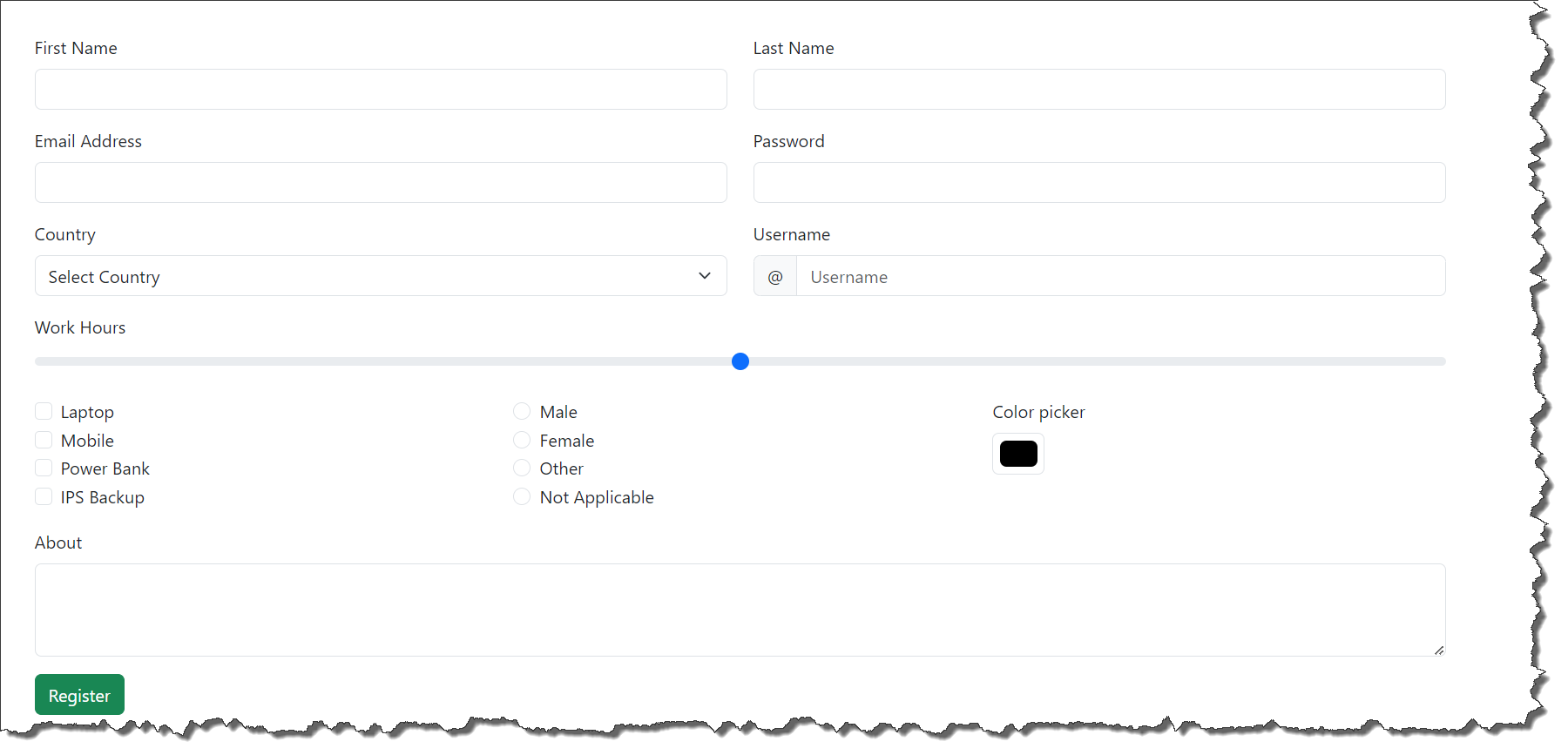